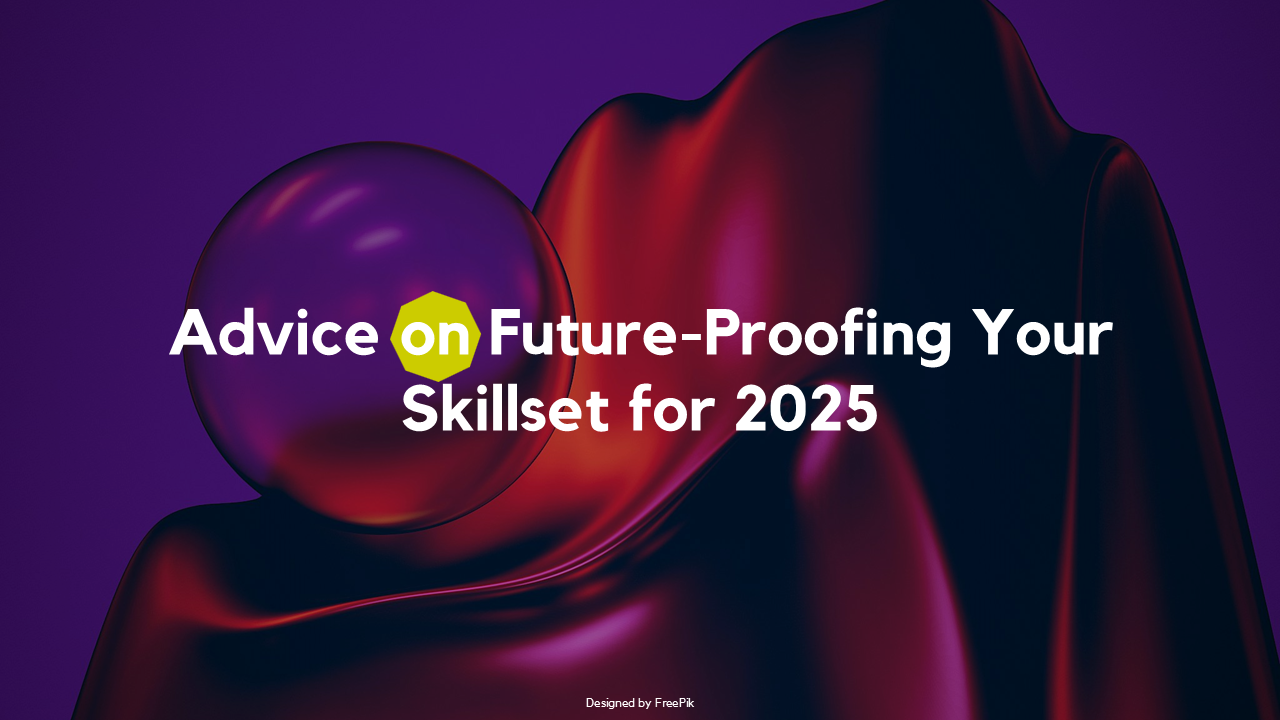
Top Interview Questions for JavaScript, .NET, & TypeScript Developers
18 Jun, 20248 minutesThe landscape of web development is constantly evolving, with new frameworks and languages e...
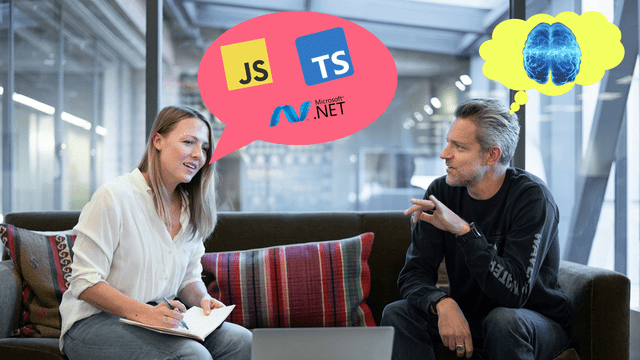
The landscape of web development is constantly evolving, with new frameworks and languages emerging all the time. When it comes to hiring developers, especially for roles involving JavaScript, .NET, or TypeScript, it's crucial to assess not just technical skills, but also cultural fit and problem-solving approach.
Finding the ideal developer for your project goes beyond just technical skills. Sure, the specific questions will vary depending on your needs (e.g., building a mobile app requires a different skillset than a back-end server). However, this blog focuses on three popular areas – JavaScript, .NET, and TypeScript – to help you identify the right cultural and technical fit for your team.
This blog delves into the world of developer interviews, providing a range of questions tailored to JavaScript, .NET, and TypeScript positions. By asking the right questions, you can ensure you find the developer who perfectly complements your team and propels your project forward.
Unveiling the Gems: Core Concepts for All Roles
Before diving into specific languages, let's explore some fundamental interview questions you should be asking that apply to all developers, regardless of their primary language:
Company Culture: How well does that candidate understand your company culture and values. Do they express a genuine interest in working for your organisation and is there enough crossover to ensure all parties are happy and working at their most optimal rate?
Learning Style: Explore how the candidate prefers to learn new technologies. Do they thrive on independent exploration, enjoy the dynamics of collaborative learning like pair programming, or prefer a balanced approach?
Additionally, gauge their interest in learning new technologies. Are they enthusiastic about expanding their skill set to include different programming languages or frameworks, or do they prefer to focus on a particular technology?
Many organisations are flexible with the level of experience required in a specific technology, willing to invest in training if a candidate shows potential in other key areas which can be a big plus for employees. It's important to determine if the candidate is open to such opportunities or if they are committed to working exclusively with their current expertise.
By understanding their learning style, goals, and openness to new technologies, you can better assess their ability to integrate and grow within your team's learning environment.
Communication Skills: Effective communication is vital within a development team. Assess the candidate's ability to explain technical concepts clearly, both verbally and in writing. How do they collaborate with designers, project managers, and other developers?
Problem-Solving Prowess: To assess a candidate's problem-solving prowess, present a real-world coding challenge and observe their thought process, ability to break it down, and communication of their approach. While speed is a plus, focus on how they justify their steps, revealing their critical thinking and problem-solving breakdown skills, ultimately seeking a candidate who can not only code but also think analytically and communicate effectively.
Debugging Dexterity: Provide a code snippet containing a deliberate bug. Gauge their ability to identify the issue, explain its cause, and suggest a solution.
Extra steps to consider:
- Learning Agility: The tech industry is ever-changing. Explore how the candidate stays updated on new trends and technologies. Ask them about recent development tools or libraries they've learned. While we previously discussed the importance of assessing a candidate's openness to learning new technologies, understanding their past learning experiences can also be valuable.
- Teamwork Harmony: Development thrives in collaborative environments. Uncover the candidate's experience working within a team, their communication style, and how they handle conflict resolution.
- Project Passion: Ask about the candidate's personal projects. This not only showcases their technical skills but also reveals their passion and drive for development.
With the basic top-line and universal questions now out of the way – let's dive into the more specific questions you can ask a Software Engineer in the JavaScript, .NET and Typescript space.
JavaScript: Unveiling the Front-End Mastermind
JavaScript has long been considered the king of front-end development, and for good reason with 95% of websites using it to make the user experience way better. But how do you weed out the good from the mediocre?
Here are some interview questions to unearth the perfect JavaScript developer:
1. Create a Countdown in JavaScript:
Core JavaScript Concepts: This tests the candidate's understanding of fundamental building blocks like variables, functions, conditional statements (if/else), loops (for/while), and the DOM (Document Object Model) for potentially manipulating the countdown display on the page.
Problem-Solving Approach: It evaluates their ability to break down a task into smaller steps, write clear and concise code, and potentially handle edge cases (e.g., negative numbers, reaching zero).
Browser Interaction: Depending on the implementation, it might assess their knowledge of the setInterval function for executing code repeatedly and updating the countdown visually.
2: Truncate a String in JavaScript:
String Manipulation: This probes the candidate's grasp of string methods like substring, slice, or potentially regular expressions for more advanced truncation.
Functionality: It gauges their ability to create reusable code that can handle strings of varying lengths.
Customisation: It might reveal their understanding of optional parameters to control truncation length or the addition of an ellipsis.
3: Implement a Resumable Interval Object:
Advanced JavaScript Concepts: This delves into a more complex topic, testing the candidate's knowledge of creating custom objects, managing state (whether using a Boolean flag or a separate property), and potentially leveraging clear Interval to pause and resume the interval.
Code Reusability: It assesses their ability to design code that can be easily reused in different parts of an application where resumable intervals might be needed.
Error Handling: It could uncover their approach to handling potential errors or unexpected behaviour, such as resetting the interval duration if it's paused for a long time.
4. Implement a Function that Serializes a JavaScript Value into a JSON String:
Data Handling: This evaluates the candidate's comprehension of data structures and the importance of data interchange formats like JSON.
Function Creation: It tests their ability to write well-structured functions that handle different JavaScript data types (numbers, strings, objects, arrays) and convert them to their corresponding JSON representations.
Edge Cases: The interview could explore how they would handle complex data structures like nested objects or circular references (objects referencing themselves).
.NET: Building Back-End Brawn
The .NET Framework has been a powerful platform for building back-end applications since its initial release (.NET 1.0 and .NET 2.0). Its importance in software development has only grown with each iteration, and it currently holds a significant share (over 25%) of the development landscape. As a result, finding skilled .NET developers can include a careful selection of questions that can assess skill.
1: Demystifying Constructors in C#:
- Importance: Grasping different constructor types (default, parameterised, static, copy, private) is crucial for creating robust C# objects. It reflects the candidate's understanding of class initialisation, memory management, and object lifecycles.
2: Managed vs. Unmanaged Code: Understanding the Divide
- Importance: Distinguishing managed and unmanaged code is essential. Managed code leverages the .NET runtime (CLR) for services like garbage collection and type safety. Unmanaged code runs directly on the OS, requiring manual memory management.
- Key Points: Evaluate their understanding of both managed and unmanaged code, including interoperability (interaction between the two).
3: WebAPI's Powerhouse: Generic Actions
- Importance: Generic actions in WebAPI enable flexible and reusable endpoints. This question assesses their grasp of ASP.NET Core's routing and controller capabilities, along with their ability to design scalable APIs.
- Key Points: Look for their knowledge of implementing generic controllers/actions, routing with constraints, data binding/validation, and use cases for generics in WebAPI.
4: Securing ASP.NET Applications: A Must-Have Skill
- Importance: Security is paramount. ASP.NET offers built-in features to safeguard applications from common threats like SQL injection, XSS, and CSRF. This question gauges their knowledge of these security mechanisms and their practical application.
- Key Points: Assess their understanding of authentication, authorisation, anti-forgery tokens, data encryption/hashing, secure configuration, and input validation.
5: Taking Control: Garbage Collection in .NET
- Importance: Understanding garbage collection (GC) is vital for efficient memory management. While the CLR handles most of it automatically, developers might need to trigger it manually in specific scenarios.
- Key Points: Evaluate their knowledge of using GC.Collect(), GC tuning, best practices, memory management concepts (generations), and memory allocation/deallocation.
6: Beautifying Applications: Themes in ASP.NET
- Importance: Applying themes in ASP.NET ensures a consistent and customisable user interface. This question assesses their understanding of ASP.NET theming and their ability to implement and manage UI styles.
- Key Points: Look for their knowledge of themes and skins, integration with CSS and master pages, dynamic theming, best practices, and practical examples of defining and applying themes.
By posing these questions, you can effectively assess a candidate's technical depth in .NET and ASP.NET, their problem-solving abilities, their awareness of best practices, and their preparedness for real-world development challenges.
TypeScript: Bridging the Gap
TypeScript offers a superset of JavaScript with static typing capabilities. Here are some questions to unearth a skilled TypeScript Software developer:
1: Advanced Type Manipulation: These questions show the developer's ability to handle complex type structures, making code more reusable and adaptable.
2: Grasp of Static Typing: Understanding static typing is crucial for using TypeScript effectively. It catches errors early, preventing crashes and making code cleaner.
3: Weighing TypeScript's Advantages: This question probes the developer's experience and their reasoning behind using TypeScript over plain JavaScript.
4: Using Type Assertions Cautiously: While TypeScript excels in type safety, these questions assess if the developer understands when and how (cautiously) to use type assertions.
By asking about these concepts, you can gauge the developer's comfort level with advanced features, their core understanding of TypeScript principles, and their ability to make sound judgments when using these features.
Beyond the Technical: Unveiling the Ideal Fit
In conclusion, finding the ideal developer for your team involves a balanced approach that assesses both technical expertise and cultural fit. In the dynamic and ever-evolving landscape of web development, this dual focus is essential, particularly for roles centred around JavaScript, .NET, and TypeScript.
Whilst this blog focuses on the overall questions you can ask Developers knowing exactly what kind of tech is needed for your project can be equally as challenging... but don't worry... we've got you covered. We've compiled a Back-End Blueprint of the the essential tech you should be looking for when hiring back-end developers and Full-Stack Developers